BLOG
Mockito package
The Mockito package in Dart is an incredibly useful tool for developers who want to create unit tests in a simple and efficient way. This library is designed to isolate the components being tested and simulate interactions with their dependencies, allowing for a thorough examination of their behavior in various scenarios.
Mockito enables easy creation of mocks for interfaces and classes, which in turn allows for testing code units without the need to rely on actual implementations. This way, developers can focus on the logic being tested, eliminating the influence of external dependencies.
Within the package, developers have the ability to define the behavior of mocks in response to method calls, enabling the simulation of different scenarios. It is easy to specify what should happen when a given method is called with specific arguments.
Additionally, Mockito allows for the verification of whether specific methods have been called, as well as the order in which they were called. This is an extremely important tool for confirming that the application logic functions as intended and that the various components work together correctly.
The Mockito package integrates seamlessly with popular testing frameworks in Dart, such as test
, which simplifies the creation, organization, and execution of unit tests. With these capabilities, Mockito becomes an invaluable asset in the process of ensuring code quality.
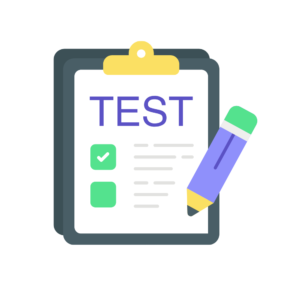
1 Set up Mockito
First, add mockito
and build_runner
to your pubspec.yaml
under dev_dependencies
:
2. Define an Abstract Class
3. Generate the Mock Class
Run the command to generate a mock class using build_runner
. Build_runner
is responsible for generating code for mock classes, also known as mocks.
4. Write a Test with mockito
Mock Instance Creation:
final mockService = MockChemicalService();
creates an instance of the mocked service.
Stub the Method: when(mockService.getMolecularWeight('H2O')).thenAnswer((_) async => 18.015);
specifies that when getMolecularWeight('H2O')
is called, it should return 18.015
.
Verify the Result:
The expect
function checks if getMolecularWeight
returned the expected value for 'H2O'
.
Verify Method Calls: verify(mockService.getMolecularWeight('H2O')).called(1);
ensures that getMolecularWeight
was called exactly once with the argument 'H2O'
.
